Using a 10-segment Led Bar with Raspberry PI and Python
Last Updated on 13th April 2024 by peppe8o
In this tutorial, I’m going to show you how to wire and use a common 10-segment LED bar with Raspberry PI.
10-segment LED bars are commonly used to get visual indicators, usually to measure filling status. You can use these electronic items with Raspberry PI, switching each LED separately
How 10 Segment LED Bar Work
The LED bar is composed simply of 10 LEDs put in a single row. Each LED can be switched on and off independently from the other.
While its front clearly shows available LED, its backside exposes the 20 pins used to drive LED status. Each LED has 2 pins, which are to be connected to positive and negative sources.
Unfortunately, there’s no clear way to identify what side goes to ground or Vcc (3V). From what I experienced with mine, the side with the label goes to Raspberry PI GPIOs which will drive on-off single LEDs, while the other side goes to Vcc.
The internal circuit can be schematized as in the following picture:
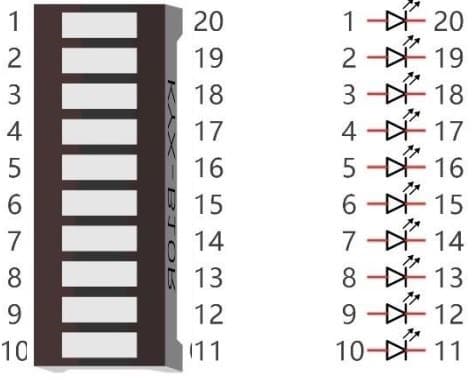
10-segment Led bars are used in many applications, the most common of which is indicating the filling level of whatever you want (water tank, battery charge, signal strength and so on).
In this tutorial, I’m going to use a Raspberry PI Zero W, but this guide applies to all Raspberry PI computer boards.
What We Need
As usual, I suggest adding from now to your favourite e-commerce shopping cart all the needed hardware, so that at the end you will be able to evaluate overall costs and decide if to continue with the project or remove them from the shopping cart. So, hardware will be only:
- Raspberry PI Zero W (including proper power supply or using a smartphone micro usb charger with at least 3A) or newer Raspberry PI Board
- high speed micro SD card (at least 16 GB, at least class 10)
- a 10 segment LED bar
- dupont wiring
- resistors
- (optional) breadboard
Check hardware prices with the following links:
Wiring Diagram
Please find below my wiring schema:
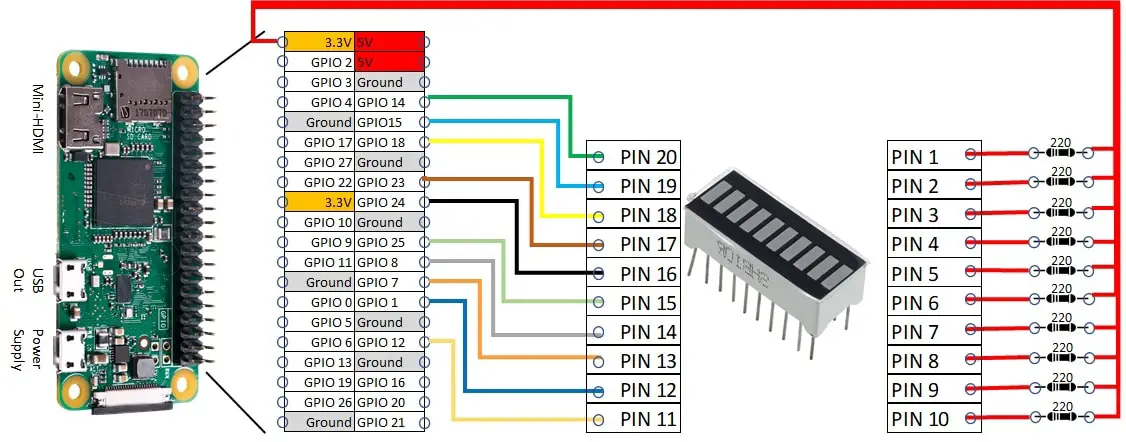
Please find below some pictures from my cabling:
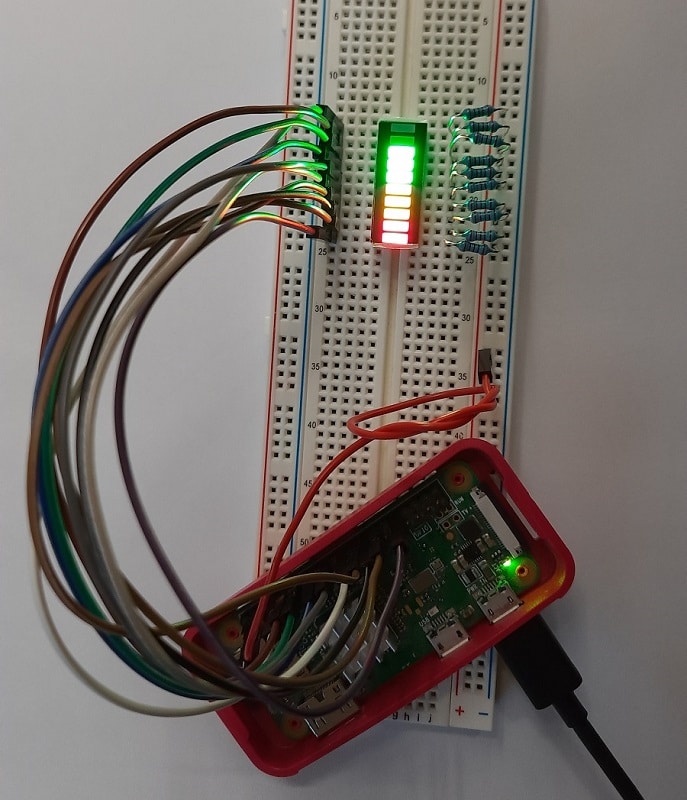
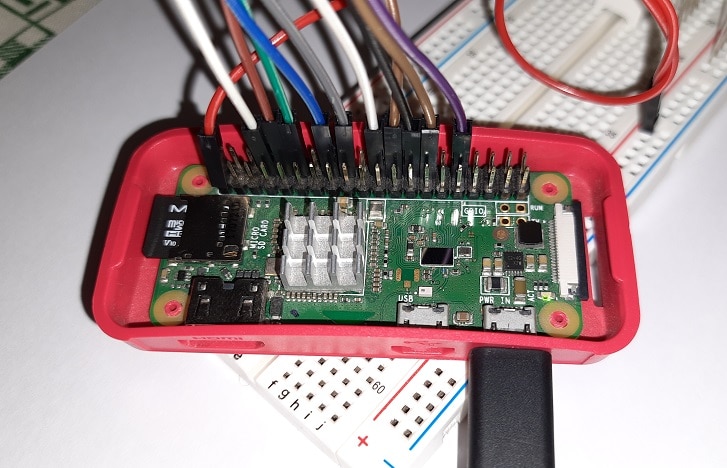
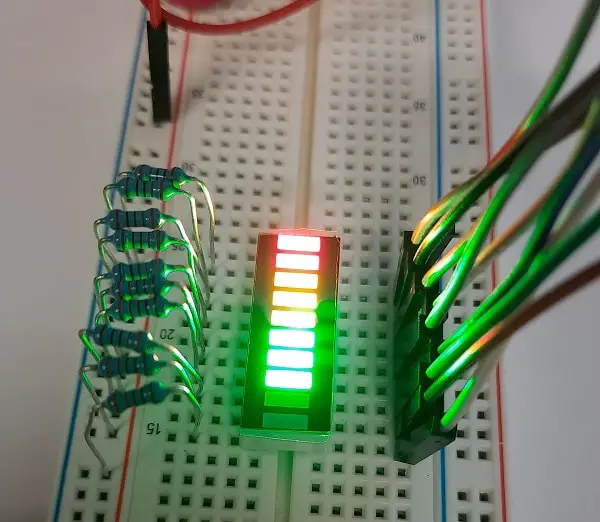
Step-By-Step Procedure
Prepare Operating system
Start installing Raspberry PI OS Lite (for a headless, fast OS) or installing Raspberry PI OS Desktop (in this case using its internal terminal).
Make your OS updated:
sudo apt update -y && sudo apt upgrade -y
RPI.GPIO should be already installed (otherwise, you can get it installed with the command “sudo apt install python3-rpi.gpio”).
Prepare 10-segment Led Bar Python Script
Going to the programming phase, you can download directly in your Raspberry PI the python script from my download area:
wget https://peppe8o.com/download/python/10SegLedBar.py
It will include 3 main functions/working modes:
- LedSegOut: showing a user-defined LED configuration
- LedSegCycle: turning LEDs on/off in a continuous loop
- LedSegPerc: showing a number of LEDs on, according to a user-defined percentage (for example, to show a filling state)
In the following paragraphs we’ll deep into code lines, before running it.
Start importing required libraries:
import sys
import time
import RPi.GPIO as GPIO
We’ll map connected GPIOs within a single variable so that we can configure all 10 segments together with the so-created array:
led_list = [14,15,18,23,24,25,8,7,1,12]
GPIOs are set to be defined with BCM (Broadcom) naming and initialized as output:
GPIO.setmode(GPIO.BCM)
for pin in led_list:
GPIO.setup(pin,GPIO.OUT)
Finally, we arrived at the functions. Our first function, named LedSegOut, requires as input a variable array, which must be an array of 10 elements (each one can be True/False or 1/0). Because of its circuit, LEDs are connected from one side to Vcc. To power on, they will need a 0 (or False) from programmable GPIOs, while they will power off when GPIO goes to 1.
The list+map+ lambda function inverts this logic so that you can work with a more comfortable association, making it possible to mark 1 as power on and 0 to power off:
- lambda in Python is a small anonymous function. Gets a variable (“n:”) and manipulates with a simple one-line function (“not(n)”). In our case, this lambda function creates the opposite of 0 or 1 values.
- map in Python applies a specified function (in its first parameter, lambda function in our script) to each single object of a given iterable (its second parameter, the array in our case)
- list function is required to convert map result (which is a map object) to a list, so that we can directly apply to the following line as output to GPIOs
def LedSegOut(array):
array=list(map(lambda n: not(n),array))
GPIO.output(led_list, array)
The result will be that if, for example, you run LedSegOut([0,0,0,0,0,1,1,1,1,1]) you will get half of LEDs powered on.
The LedSegCycle function is used to power on 1 LED at time, switching from one to the other in an infinite loop (until you press CTRL+C to trigger the except exit). It doesn’t need any input. It uses the python shift function (“array[1:]+array[:1]”) to change at each while loop iteration the selected LED. It also uses LedSegOut to make LEDs powered on.
The time sleep function defines how much time it takes to pass from one LED to the following one. The lower this delay, the faster your cycle will run:
def LedSegCycle():
array=[0,0,0,0,0,0,0,0,0,1]
while True:
try:
array = array[1:]+array[:1]
LedSegOut(array)
time.sleep(.05)
except KeyboardInterrupt:
GPIO.cleanup()
sys.exit()
LedSegPerc function works by setting an array of thresholds instead of LED status. It requires a number between 0 and 100 (so you don’t have to specify “%” character). When this function runs, it compares the number in input (“n”) to each thresholds array item. This is made compact with a ternary operator in lambda function. “(1,0)[x>n]” is a compact and fast alternative way to write “if x>n then x=1 else x=0”. So, each threshold value is changed to 0 or 1 if input is higher or lower to that threshold value. Finally, LEDs are again switched on/off by using LedSegOut function:
def LedSegPerc(n):
thresholds=[100,90,80,70,60,50,40,30,20,10]
array=list(map(lambda x: (1,0)[x>n],thresholds))
LedSegOut(array)
The main loop lets you choose which function to execute (by commenting the other 2 and leaving uncommented the function you want to use). In my script there are also examples of values you can use or edit for your very first tests:
while True:
try:
# LedSegOut([0,0,0,0,0,1,1,1,1,1])
LedSegCycle()
# LedSegPerc(81)
except KeyboardInterrupt:
GPIO.cleanup()
sys.exit()
As usual, the “except KeyboardInterrupt:” will keep your terminate command (wih keyboard CTRL+C combination) and will clean GPIOs status exiting correctly from the script.
Run Python Script
Once ready and once you have choose what function to test (uncommenting your test one and commenting with “#” char the other functions), you can run this script with following terminal command:
python3 10SegLedBar.py
And if your cabling is correct, you will see your 10 segment LED bar starting to run.
If nothing happens, please check your cabling. If cabling is ok, try changing verse to your bar as different producers could apply the label in different positions.
Enjoy!